When you download the source code from
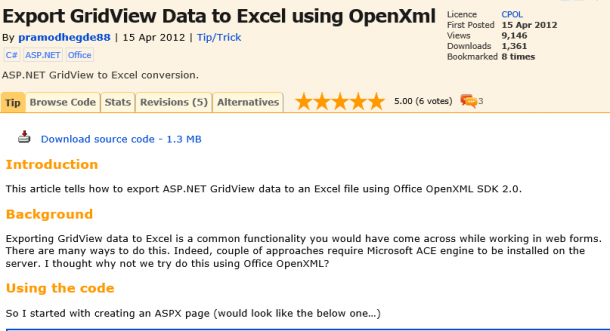
http://www.codeproject.com/Tips/366446/Export-GridView-Data-to-Excel-using-OpenXml
and try to transfer it to Excel from a single cell to another Excel file, it is not easy.
I have broken a big chunk into a smaller unit to just output one value to an Excel workbook using OpenXML SDK. Below is the part of code
segments that doing this.
//Simple
private void
CreateExcelParts(SpreadsheetDocument
spreadsheetDoc, string str)
{
//Create a Workbook
WorkbookPart workbookPart =
spreadsheetDoc.AddWorkbookPart();
CreateWorkbookPart(workbookPart);
//Create a worksheet
Worksheet worksheet = new
Worksheet() { MCAttributes = new MarkupCompatibilityAttributes()
{ Ignorable = “x14ac” } };
worksheet.AddNamespaceDeclaration(“r”,
“http://schemas.openxmlformats.org/officeDocument/2006/relationships”);
worksheet.AddNamespaceDeclaration(“mc”,
“http://schemas.openxmlformats.org/markup-compatibility/2006”);
worksheet.AddNamespaceDeclaration(“x14ac”,
“http://schemas.microsoft.com/office/spreadsheetml/2009/9/ac”);
//Create sheetviews
SheetViews sheetViews = new
SheetViews();
SheetView sheetView = new
SheetView() { WorkbookViewId = (UInt32Value)0U };
//Create a cell or range
Selection selection = new
Selection() { ActiveCell = “A1” };
//Append range to sheetview
sheetView.Append(selection);
//Append sheetview to sheetviews
sheetViews.Append(sheetView);
int columnIndex = 0;
SheetData sheetData = new
SheetData();
UInt32Value rowIndex = 1U;
//Create a row
Row row1 = new Row()
{
RowIndex
= rowIndex++,
Spans = new ListValue<StringValue>() { InnerText = “1:3” },
DyDescent
= 0.25D
};
//Create a cell and put the value in it
Cell cell = new Cell() { CellReference = ExcelHelper.ColumnCaption.Instance.Get((Convert.ToInt32((UInt32)rowIndex)
– 2), columnIndex), DataType = CellValues.String
};
CellValue cellValue = new
CellValue();
//Create a CellValue to store the value
//Assign the value to new cell
cellValue.Text = str;
//Append the cellValue into cell
cell.Append(cellValue);
//Append the cell to row
row1.Append(cell);
//Append the row to sheetdata
sheetData.Append(row1);
//Append the sheetData to worksheet
worksheet.Append(sheetData);
WorksheetPart worksheetPart =
workbookPart.AddNewPart<WorksheetPart>(“rId” + (101).ToString());
//Append the worksheet into workbook
workbookPart.WorksheetParts.ElementAt(0).Worksheet = worksheet;
//Save the workbook
workbookPart.Workbook.Save();
}
/// <summary>
/// Creates the workbook part.
/// </summary>
/// <param name=”workbookPart”>The workbook part.</param>
private void
CreateWorkbookPart(WorkbookPart
workbookPart)
{
Workbook workbook = new
Workbook();
Sheets sheets = new
Sheets();
Sheet sheet = new Sheet()
{
Name
= “Book” + 1,
SheetId = Convert.ToUInt32(101),
Id = “rId” + (101).ToString()
};
sheets.Append(sheet);
CalculationProperties calculationProperties = new CalculationProperties()
{
CalculationId = (UInt32Value)123456U // some default
Int32Value
};
workbook.Append(sheets);
workbook.Append(calculationProperties);
workbookPart.Workbook = workbook;
}
The code segment is pretty much self-explanatory.
You can also download a single solution from http://skydrive.live.com. The sample file name is GridToExcelNew.rar
If you have any questions, feel free to ping me. My MSN ID is chanmmn@hotmail.com.